Welcome to another exploration of coding challenges! Today, we will tackle a popular problem from the domain of dynamic programming: Combination Sum IV. This problem is a perfect example to understand different strategies to solve a problem and optimize it efficiently. Let’s dive right in!

If you’re just joining us, it might be helpful to catch up on the previous entries. Want to see more problem-solving techniques? Follow me here on my blog!
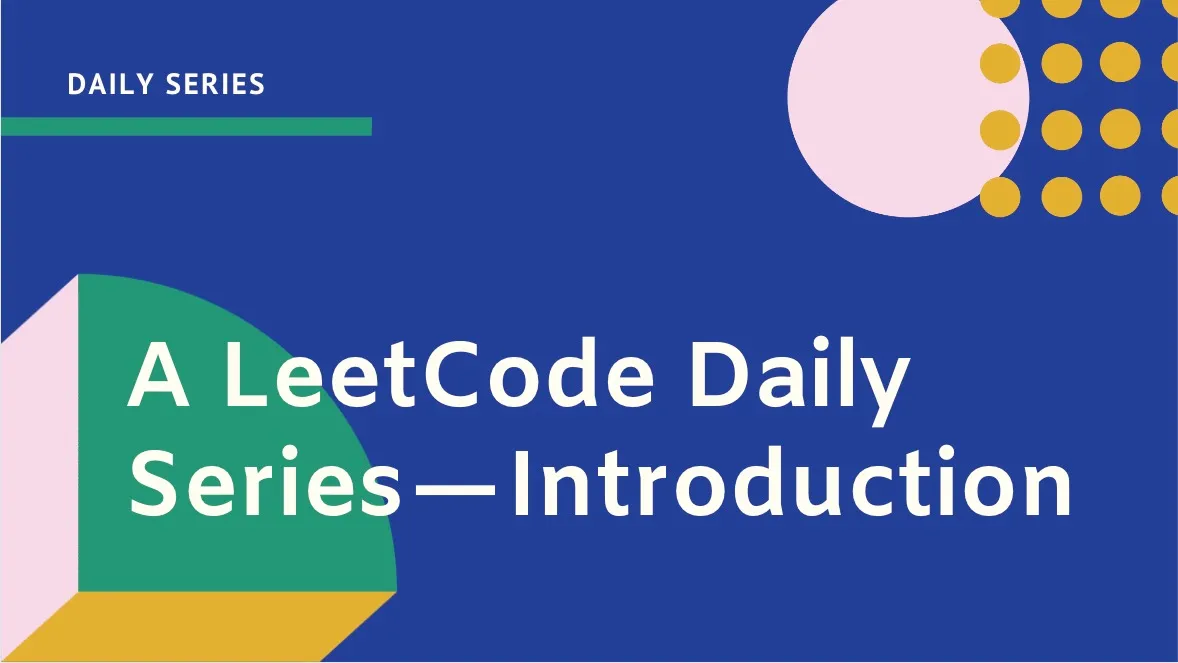
Understanding the Problem
Given an array of distinct integers nums
and a target
integer target, our goal is to return the number of possible combinations that add up to target. The combinations can use numbers from nums multiple times, and the order of elements in the combinations matters.
Example 1:
Input: nums = [1,2,3], target = 4
Output: 7
Explanation:
The possible combination ways are:
(1, 1, 1, 1)
(1, 1, 2)
(1, 2, 1)
(1, 3)
(2, 1, 1)
(2, 2)
(3, 1)
Note that different sequences are counted as different combinations.
Example 2:
Input: nums = [9], target = 3
Output: 0
Using Efficient Approach: Dynamic Programming (Bottom-Up)
A bottom-up dynamic programming approach is often useful for problems involving combinations and permutations. This method involves building a solution iteratively, starting from the smallest subproblems and using their solutions to solve larger problems.
def combinationSum4(self, nums: List[int], target: int) -> int:
dp = { 0 : 1 }
for total in range(1, target + 1):
dp[total] = 0
for n in nums:
dp[total] += dp.get(total - n, 0)
return dp[target]
Explanation
- Initialization: We create a dictionary
dp
wheredp[0] = 1
because there is one way to make the target sum0
, which is using no numbers at all. - Iterate Over Targets: We iterate from
1
to the target, calculating the number of combinations for each intermediate targettotal
. - Iterate Over Numbers: For each
total
, we iterate through all numbers innums
. If subtracting a numbern
fromtotal
results in a non-negative value, we add the number of combinations for the resulting value todp[total]
. - Return Result: Finally, we return
dp[target]
, which contains the number of combinations that sum up totarget
.
Time Complexity: O(n×target)
, where n
is the length of nums. This is because we have a nested loop: the outer loop runs target times and the inner loop runs n
times.
Space Complexity: O(target)
. We use a dictionary to store combinations for each intermediate target up to the given target.
Using Efficient Approach 2: Dynamic Programming (Top-Down with Memoization)
A top-down approach with memoization involves solving the problem recursively and storing the results of subproblems to avoid redundant computations. This is particularly effective when dealing with overlapping subproblems.
def combinationSum4(self, nums: List[int], target: int) -> int:
dp = {}
def combinationSum(nums, target):
if target == 0:
return 1
if target in dp:
return dp[target]
comb = 0
for num in nums:
if target >= num:
comb += combinationSum(nums, target - num)
dp[target] = comb
return dp[target]
return combinationSum(nums, target)
Explanation
- Memoization Dictionary: We use a dictionary
dp
to store the results of subproblems. - Base Case: If the
target
is0
, there is exactly one combination (using no elements), so we return1
. - Recursive Case: If the result for the current
target
is already computed (present indp
), we return it to avoid recomputation. - Compute Combinations: For each number in
nums
, if it is less than or equal to thetarget
, we recursively compute the combinations for the remaining target (target - num
). - Store Result: After computing, we store the result in
dp
and return it.
Time Complexity: O(n×target)
, where n
is the length of nums. The recursion ensures that each subproblem is solved once and stored.
Space Complexity: O(target)
for the memoization dictionary dp
plus the recursive call stack.
Conclusion
In this article, we explored the Combination Sum IV problem and discussed two efficient dynamic programming approaches to solve it. By comparing these methods, we understand the importance of optimizing our code for better performance and scalability.
Each approach has its trade-offs in terms of time and space complexity, and choosing the right approach depends on the constraints of the problem and the size of the input.
Found this helpful? Follow me for more leetcode adventures! Questions? React out via email.